API Rate Limiting
API Rate Limiting is used to limit the number of requests a client can make to an API.
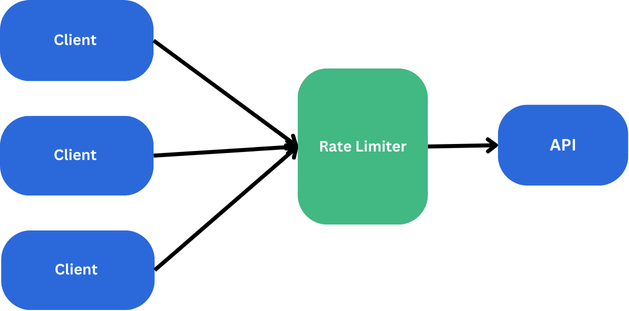
There are different rate limiting algorithms such as the Fixed Window algorithm. Let's see this algorithm in action.
- Rate limiting is setup such that a client can make 10 requests per hour to the API
- The client makes 10 requests in the first 15 minutes
- Client is blocked from making any further requests to the API for 45 minutes
After the fixed time window is up, the client can continue to send requests to the API again. Rate limiting protects against API misuse, DoS attacks, web scraping, etc.
ASP.NET Core provides rate limiting middleware out of the box. Let's implement a minimal API using Microsoft.AspNetCore.RateLimiting middleware. We will use the sample Weather Forecast ASP.NET Core Web API application made available by Microsoft. This app provides an API that returns a list of randomized weather forecast data.
using Microsoft.AspNetCore.RateLimiting;
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddRateLimiter(_ => _
.AddFixedWindowLimiter(policyName: "fixed", options =>
{
options.PermitLimit = 5;
options.Window = TimeSpan.FromSeconds(10);
}));
var app = builder.Build();
app.UseRateLimiter();
var summaries = new[]
{
"Freezing", "Bracing", "Chilly", "Cool", "Mild", "Warm", "Balmy", "Hot", "Sweltering", "Scorching"
};
app.MapGet("/weatherforecast", () =>
{
var forecast = Enumerable.Range(1, 5).Select(index =>
new WeatherForecast
(
DateOnly.FromDateTime(DateTime.Now.AddDays(index)),
Random.Shared.Next(-20, 55),
summaries[Random.Shared.Next(summaries.Length)]
))
.ToArray();
return forecast;
}).RequireRateLimiting("fixed");
app.Run();
internal record WeatherForecast(DateOnly Date, int TemperatureC, string? Summary)
{
public int TemperatureF => 32 + (int)(TemperatureC / 0.5556);
}
The preceding code creates a fixed window limiter with a PermitLimit of 5 and Window of 10 seconds. That is a maximum of 5 requests can be made by a client per each 10 second window.