Cache REST Constraint
There are 6 REST architectural constraints.
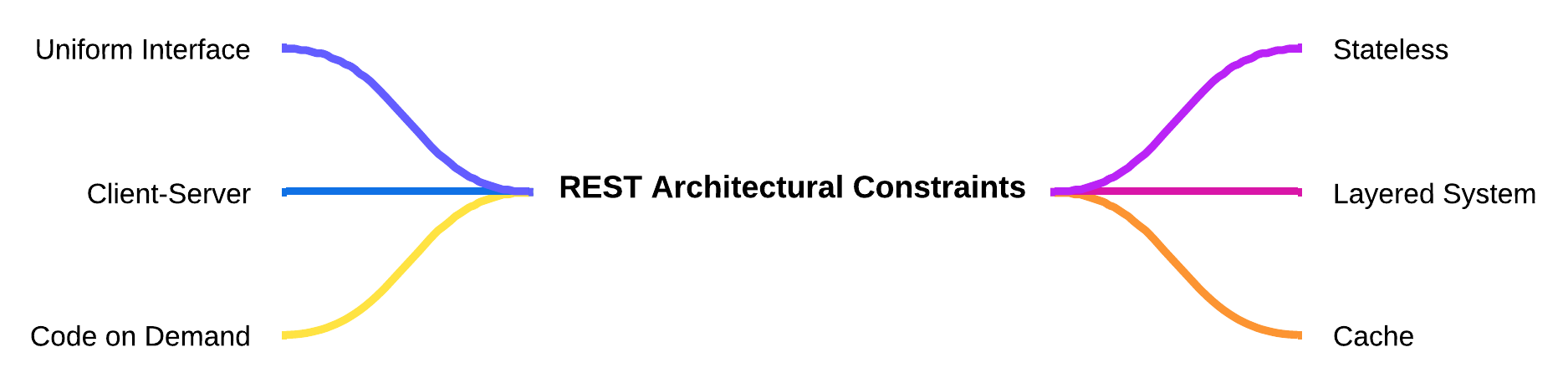
Let's talk about the Cache constraint in detail. Roy Fielding (the guy who invented REST), defined this constraint in his dissertation as:
Cache constraints require that the data within a response to a request be implicitly or explicitly labeled as cacheable or non-cacheable.
In other words, every time a response is returned from the API to the client, the API must explicitly state if it can be cached or not. If it can be cached, then the client can use the cached data instead of calling the API again hence improving performance.
But how do we know if a response from the API can be cached or not?
Let's send a request to an API to get the current Bitcoin price. This is CoinDesk's public API.
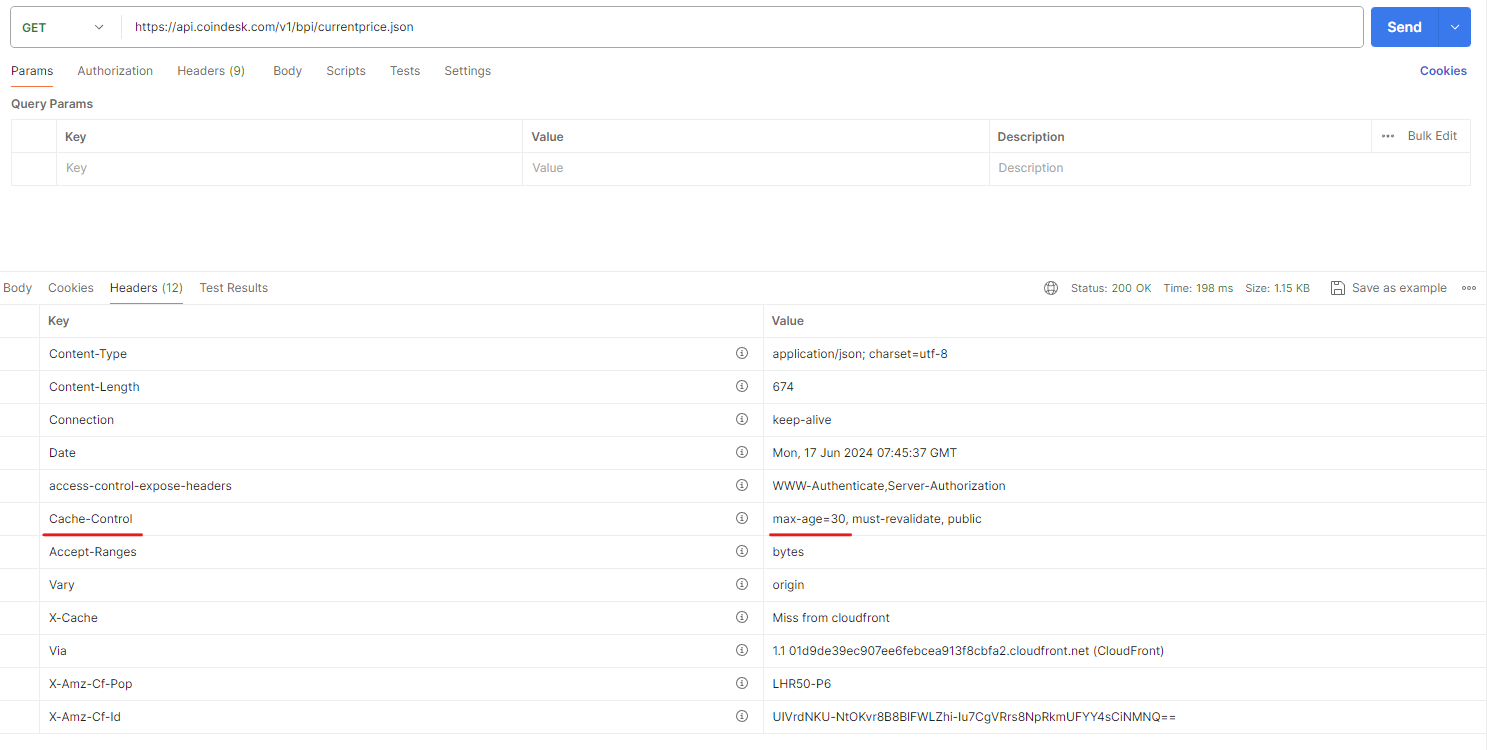
By looking at response headers, you can see a Cache-Control header which indicates that this response will remain fresh for 30 seconds. After 30 seconds the response is considered stale and a new request to the API will need to be made.
Cache-Control is just one cache related response header. Other cache related headers include Expires, Age, etc, all of which can be found on the Mozilla Developer Network.
Let's take a look at how we can add a Cache-Control field to the response header and cache responses in ASP.NET Core Web API. We will use the sample Weather Forecast API application made available by Microsoft. This app provides an API that returns a list of randomized weather forecast data. To add a Cache-Control field to the response header we can use the ResponseCache attribute.
[HttpGet]
[ResponseCache(Duration = 30)]
public IActionResult Get()
{
var weatherForecast = Enumerable.Range(1, 5).Select(index => new
WeatherForecast
{
Date = DateTime.Now.AddDays(index),
TemperatureC = Random.Shared.Next(-20, 55),
Summary = Summaries[Random.Shared.Next(Summaries.Length)]
}).ToArray();
return Ok(weatherForecast);
}
We also need to add the ResponseCaching service to the container and to the request pipeline.
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddControllers();
builder.Services.AddResponseCaching();
var app = builder.Build();
app.UseResponseCaching();
app.UseAuthorization();
app.MapControllers();
app.Run();
Now if the API is invoked the response header contains the Cache-Control field with a max-age value of 30 meaning that this response will remain fresh for 30 seconds. After 30 seconds the response is considered stale and a new request to the API will need to be made.
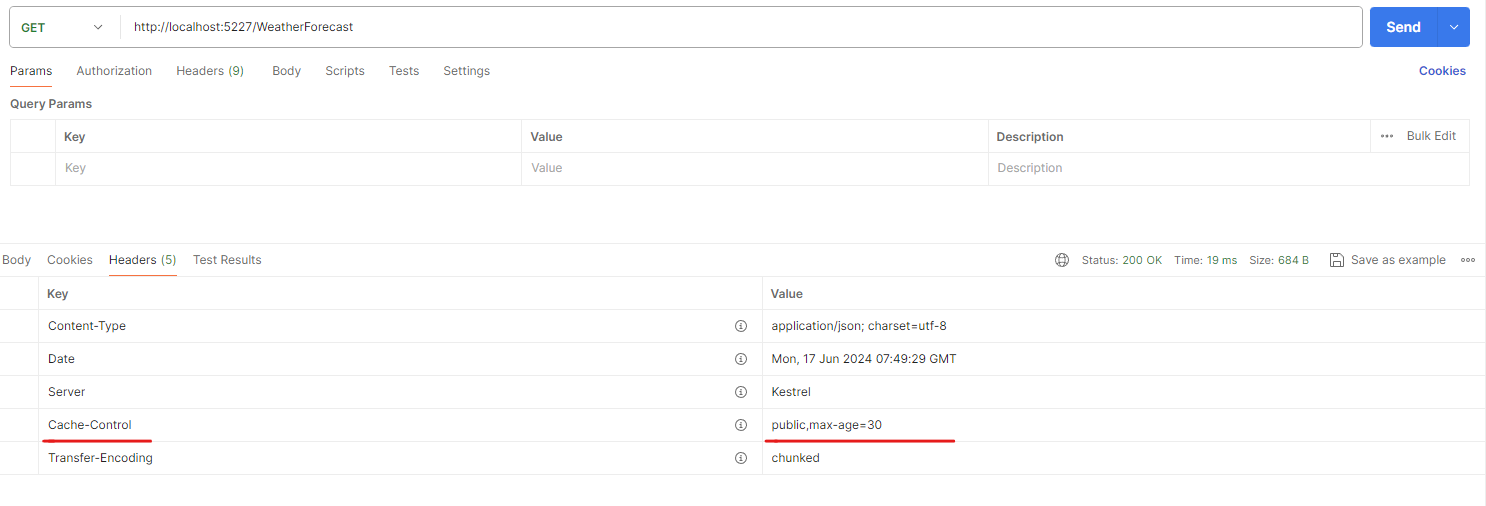