Don't PUT it, PATCH it
There are two options for updating resources:
- PUT - used for full updates
- PATCH - used for partial updates
What is the difference between full and partial updates?
Consider a social media platform like Instagram that offers an API to allow users to update their profile details (e.g. name, location, etc).
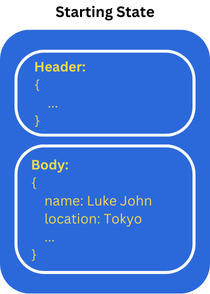
Sending a PUT request to this API will override all of the resource fields (the name and location) even though we only require the location to be updated from Tokyo to New York.
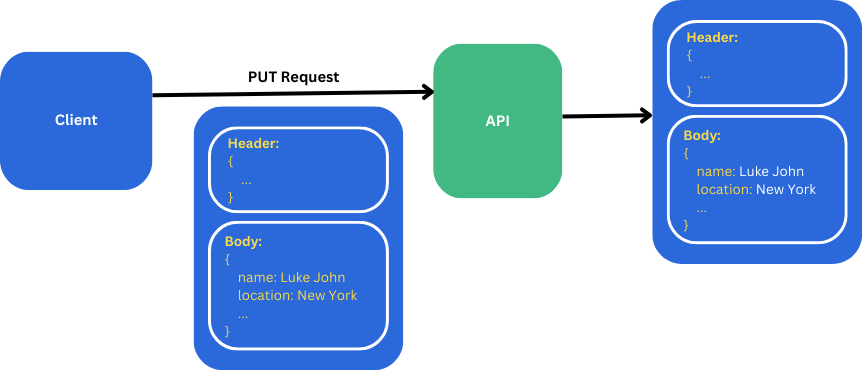
Sending a PATCH request to the API though would not override all of the resource fields but only the relevant fields (e.g. the location only and not the name).
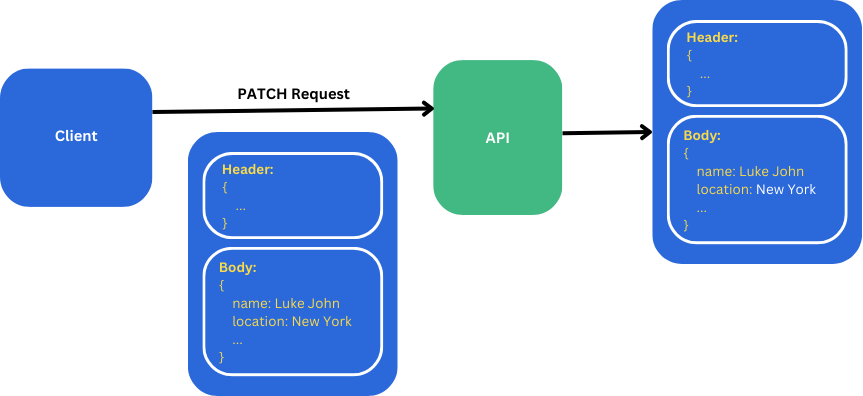
There are performance implications when it comes to using PUT for updates. In this example we are only sending the name and location in the request.
What if we had thousands of fields to send? This would be very inefficient and using PATCH would massively improve performance since we only send fields that need updating.
The request payload for PATCH is in the form of a JsonPatchDocument. This specifies a few things:
- op - the operation to be performed on the property of the resource (e.g. add, remove, replace, etc)
- path - the path to the property of the resource
- value - the new value of the property
You can find more details on JsonPatch.com
Let's implement a PATCH API endpoint using the ASP.NET Core implementation of JSON Patch - the Microsoft.AspNetCore.JsonPatch NuGet package.
Here's the action method for API endpoint:
[HttpPatch("{id}")]
public IActionResult Patch(Guid id, [FromBody] JsonPatchDocument<UserProfile> patchDoc)
{
var profileToUpdate = _userProfiles.FirstOrDefault(f => f.Id == id);
if (profileToUpdate == null) return NotFound();
patchDoc.ApplyTo(profileToUpdate);
return Ok(profileToUpdate);
}
The action method:
- Accepts a JsonPatchDocument from the request body
- Finds the object that needs to be updated. This would come from a database but for this demo, we are using in-memory objects
- Calls the ApplyTo method on the patch document passing in the in-memory object we want to update. We are updating the location from Tokyo to New York.
When invoking this API endpoint with Postman, we specify the Content-Type value with application/json-patch+json.
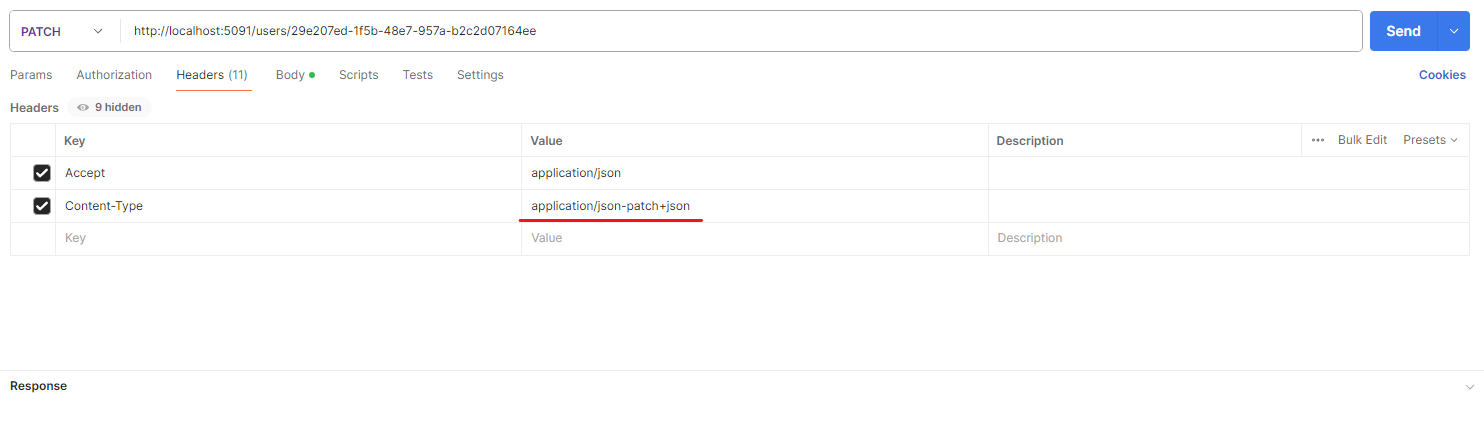
We also provide the JSON Patch request in the request body and the updated resource is returned.
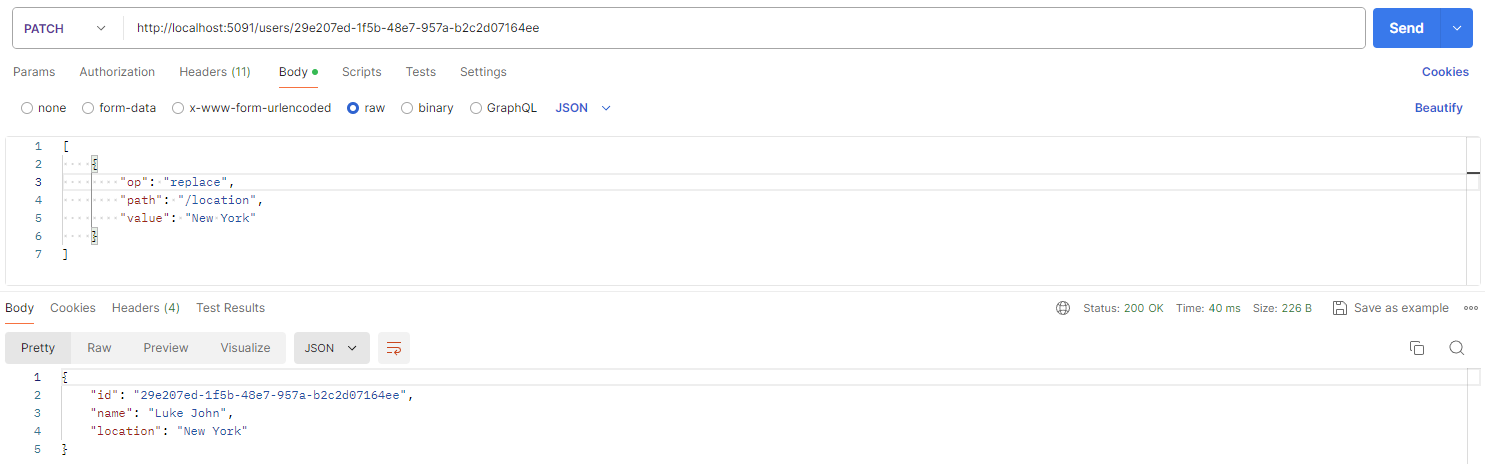
This is a simple app. A real world app would be much more complex, retrieving the data from a database, applying the patch and then updating the database.