Load Balancing using YARP Reverse Proxy
In this blog post, we will be using YARP (Yet Another Reverse Proxy) as a load balancer to forward user requests to the appropriate backend servers.
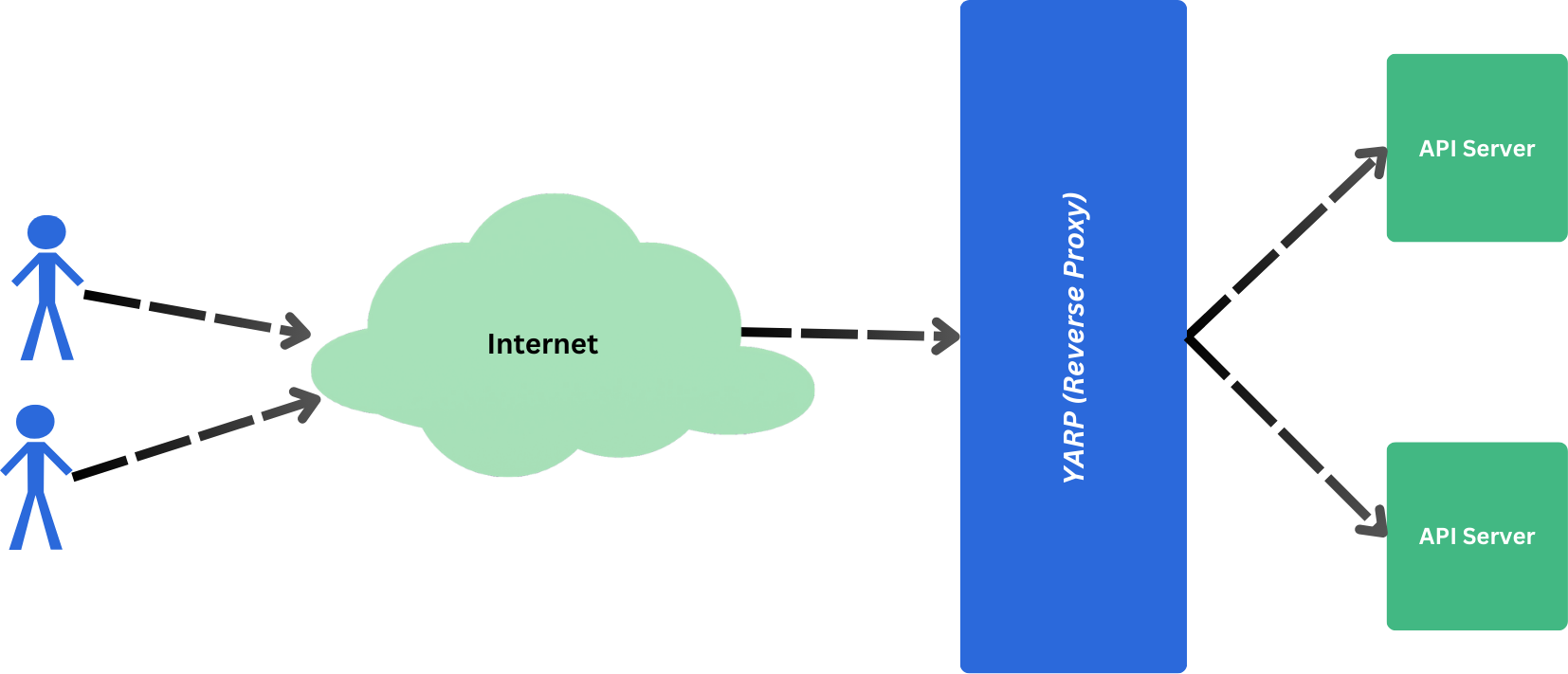
Let's go through the code. There are two ASP.NET Core Minimal APIs.
API 1 returns Hello World from API 1 when the GET request is invoked. The service is available on: https://localhost:7100/
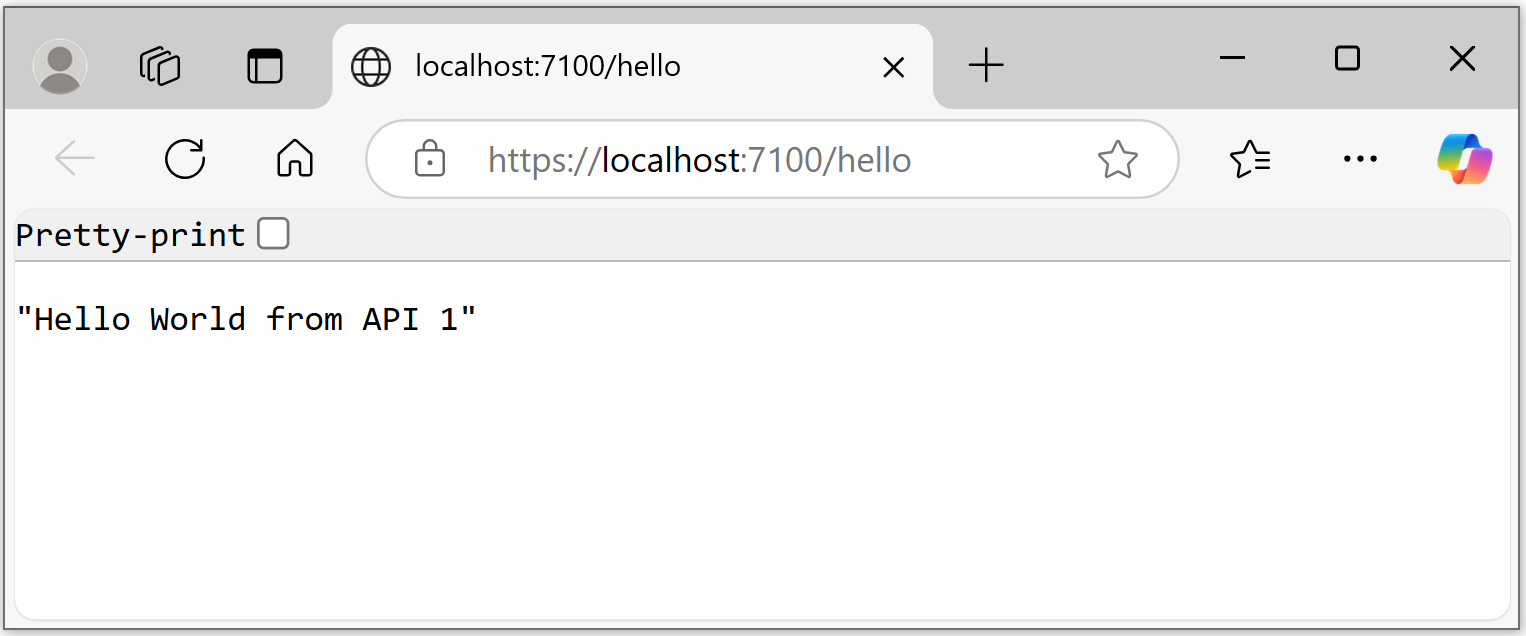
API 2 returns Hello World from API 2 when the GET request is invoked. The service is available on: https://localhost:7200/
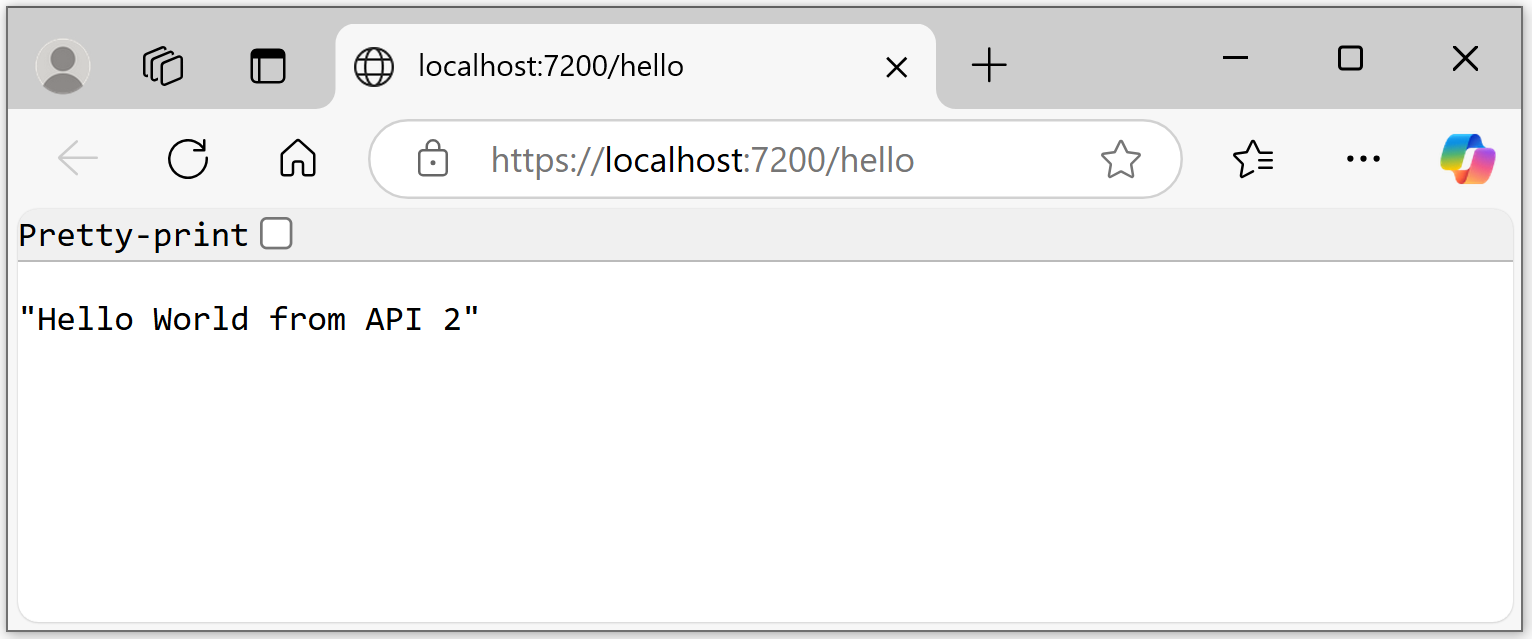
The reverse proxy (YARP) is a separate ASP.NET Core Minimal API that forwards user requests to API 1 and API 2. The reverse proxy is available on this address: https://localhost:7300/
YARP is a library developed by Microsoft. First step is to install it via the NuGet Package Manager.
Install-Package Yarp.ReverseProxy
Next step is to modify Program.cs
to make use of YARP.
var builder = WebApplication.CreateBuilder(args);
builder.Services.AddReverseProxy()
.LoadFromConfig(builder.Configuration.GetSection("ReverseProxy"));
var app = builder.Build();
app.MapGet("/", () => Results.Ok("Hello from YARP"));
app.MapReverseProxy();
app.Run();
AddReverseProxy
- Adds the YARP serviceLoadFromConfig
- Loads configuration settings from the ReverseProxy section of appsettings.jsonMapReverseProxy
- Adds reverse proxy middleware
We now need to tell YARP how to route incoming requests to the backend servers. These details are defined in appsettings.json under the Routes and Clusters sections.
{
"Logging": {
"LogLevel": {
"Default": "Information",
"Microsoft.AspNetCore": "Warning"
}
},
"AllowedHosts": "*",
"ReverseProxy": {
"Routes": {
"api-route": {
"ClusterId": "api-cluster",
"Match": {
"Path": "/hello"
}
}
},
"Clusters": {
"api-cluster": {
"LoadBalancingPolicy": "RoundRobin",
"Destinations": {
"api1-destination": {
"Address": "https://localhost:7100/"
},
"api2-destination": {
"Address": "https://localhost:7200/"
}
}
}
}
}
}
Requests to /hello
are forwarded to two backend servers (https://localhost:7100/
and https://localhost:7200/
) using round-robin load balancing.
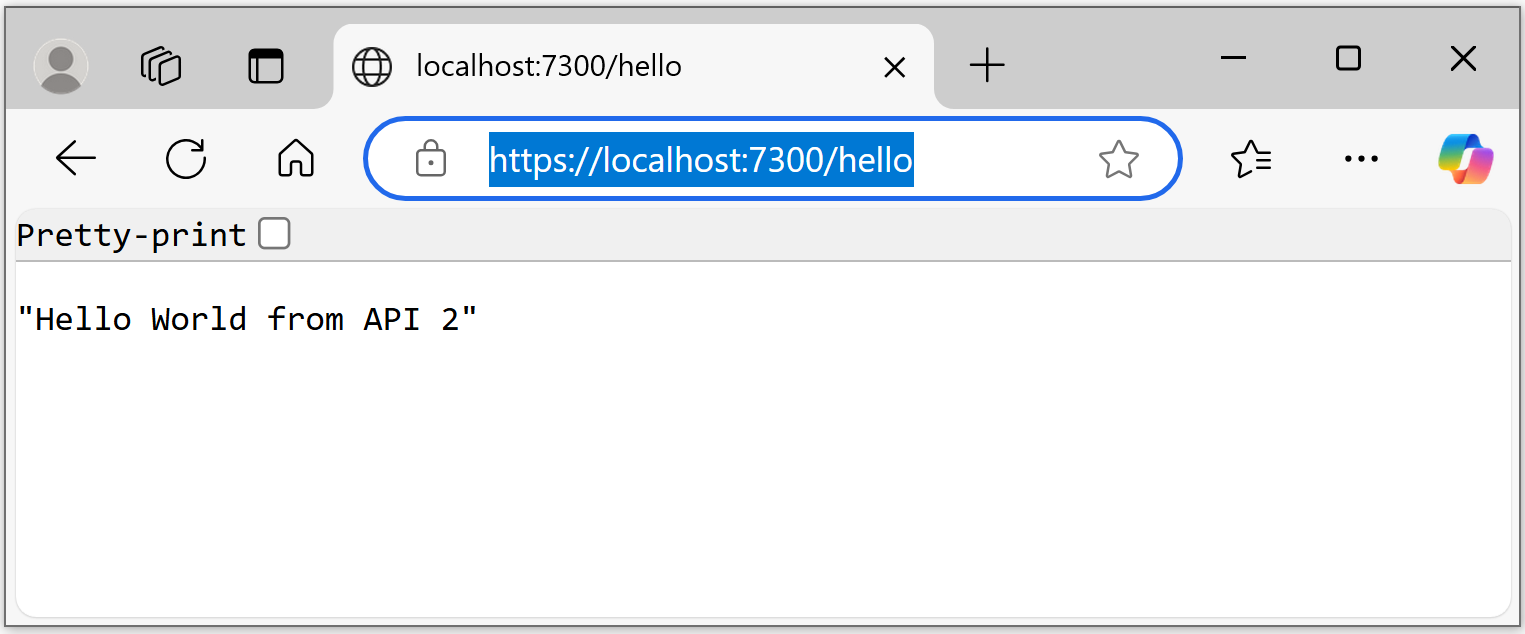
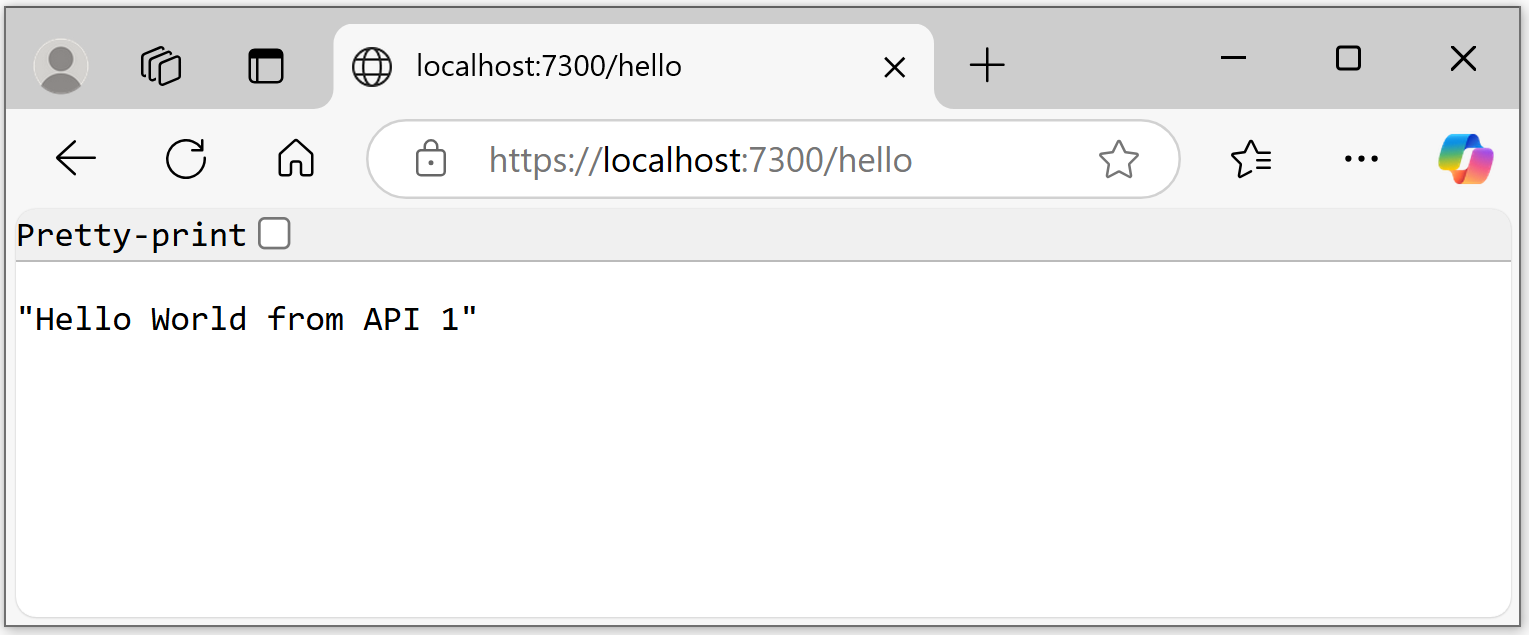