Why is API Versioning Important?
An API can change in many different ways. For example, a property could be removed on the response. Regardless of whatever the API change is, it should never break the consumers of the API.
Let's first take a look at an example of a working API and a client that consumes the API. We will then remove a property on the response of the API breaking the client. Finally we will use URI Versioning to maintain compatibility between the API and its client.
Working API and Client
We will use the sample Weather Forecast ASP.NET Core Web API application made available by Microsoft. This app provides an API that returns a list of randomized weather forecast data. The client is a Vue.js app that consumes the API by making a HTTP GET call and displaying the list of weather forecast data in the UI.
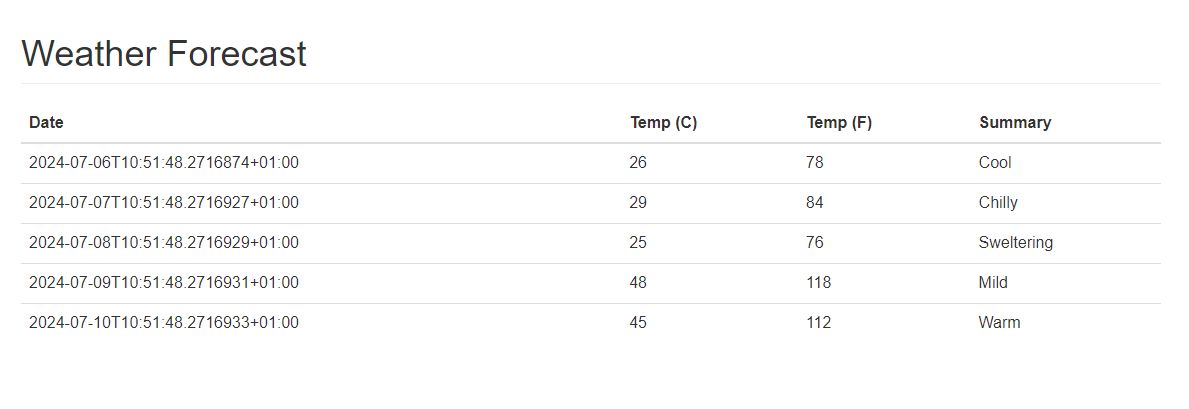
In this scenario the API and client are working as expected i.e. we have not introduced a breaking change in the API that then breaks the client consuming it.
Introducing a Breaking API Change
Let's now introduce a breaking change in the API that will then also break the client. We will remove the Summary on the response.
[HttpGet]
[Route("[action]")]
public IEnumerable Get()
{
return Enumerable.Range(1, 5).Select(index => new WeatherForecast
{
Date = DateTime.Now.AddDays(index),
TemperatureC = Random.Shared.Next(-20, 55)
})
.ToArray();
}
Prior to this change, the Summary property was being consumed by the client.
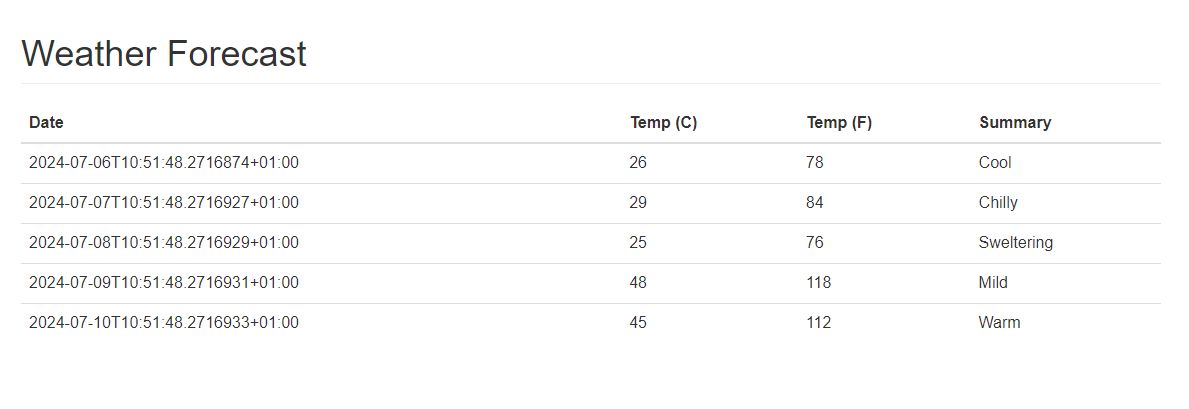
But now there is no summary data in the response and hence the API change has introduced a breaking change on the client.
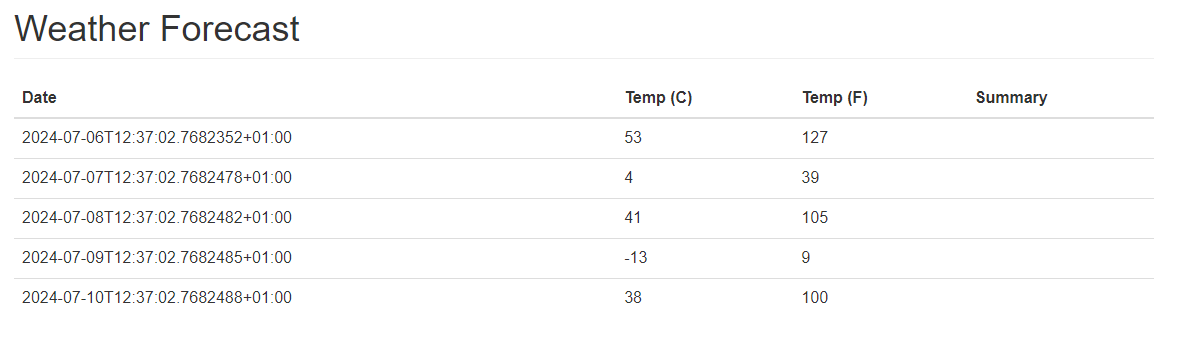
How can we roll out API changes successfully whilst ensuring we do not break clients?
We can use API Versioning. We can create and manage different versions of the API ensuring backwards compatibility. This way clients can choose which version of the API to consume. In this case, we will use URI Versioning. There are other types of API Versioning methods such as Query Parameter Versioning, Header Versioning, etc.
URI Versioning to the Rescue
With URI Versioning, the version number is included in the URI path. In the context of the Weather Forecast API, the client would specify the version of the API to be consumed.
http://localhost:5099/api/v1/WeatherForecast/Get
http://localhost:5099/api/v2/WeatherForecast/GetV2
http://localhost:5099/api/v1/WeatherForecast/Get is to be used by clients who require the Summary in the response.
[HttpGet, MapToApiVersion("1")]
[Route("[action]")]
public IEnumerable Get()
{
return Enumerable.Range(1, 5).Select(index => new WeatherForecast
{
Date = DateTime.Now.AddDays(index),
TemperatureC = Random.Shared.Next(-20, 55),
Summary = Summaries[Random.Shared.Next(Summaries.Length)]
})
.ToArray();
}
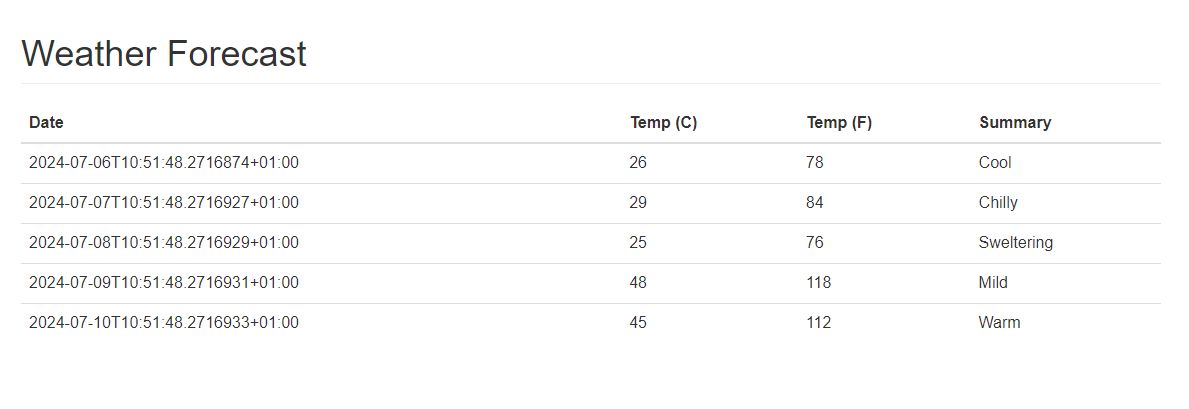
is to be used by clients who do not require the Summary in the response.
[HttpGet, MapToApiVersion("2")]
[Route("[action]")]
public IEnumerable GetV2()
{
return Enumerable.Range(1, 5).Select(index => new WeatherForecast
{
Date = DateTime.Now.AddDays(index),
TemperatureC = Random.Shared.Next(-20, 55)
})
.ToArray();
}
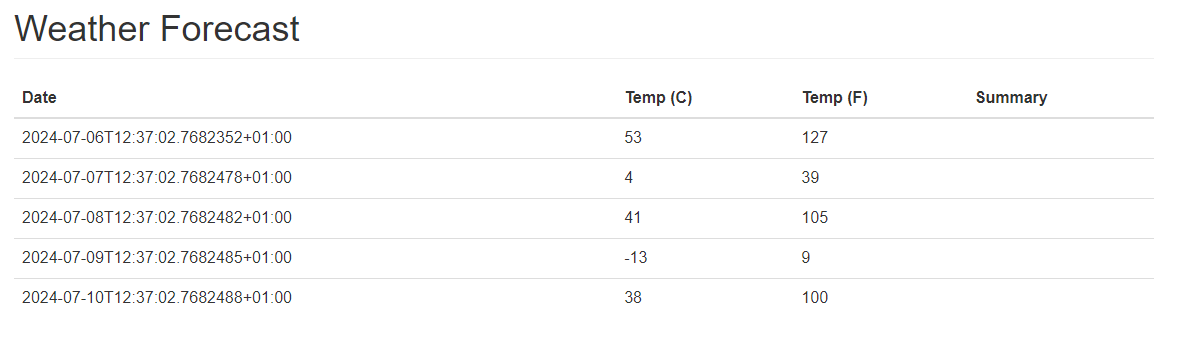
Using API Versioning will allow the API to evolve while maintaining backwards compatibility.